Getting Started
This guide provides an introduction to getting started with the Harbor API, including how to authenticate requests, create customers, link blockchain addresses or bank accounts, and make API calls with the required headers and parameters.
Role Definitions
- Harbor: Refers to us in this document.
- Application: Our integration partners.
- Customer: Typically the customers of an Application.
Due to compliance requirements, Harbor requires Applications to provide Customer KYC (Know Your Customer) information during integration. Alternatively, we provide a direct KYC link that enables customers to submit their information seamlessly.
You are also the payer
If you are a application that also wants to perform payments, please onboard yourself as a Customer and pass the parameters to
business
to get the KYB form.
Once a Customer submits their KYC data, the Application can refer to the following status flow diagram to understand the current review status. For a detailed explanation of each status, please refer to Status .
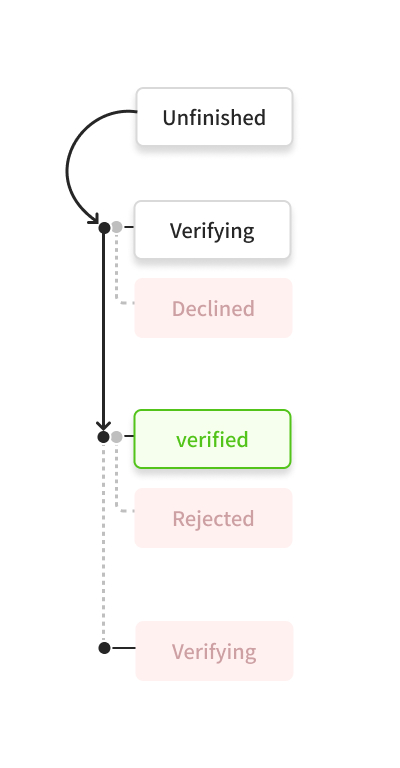
Customer KYC status change diagram
Step 1: Contact us to get Sandbox/Production information
To get started with OwlPay Harbor, please contact us to obtain sandbox and production access. We'll first provide you with sandbox credentials and an API_KEY for testing in your environment. Once you've completed the integration, we'll issue your PRODUCTION API_KEY, allowing you to go live 🎊.
Keep your API KEY safe
If you lose your PRODUCTION API_KEY, contact our customer service immediately. Ensure that your API_KEY is stored securely and never shared or exposed to insecure environments to prevent potential leaks.
Step 2: Register Your First Customer
You are now ready to start using Harbor’s core APIs.
The first step is to create a customer object that represents a user in your system.
When making this request, you need to set specific headers as shown in the example below. Most importantly, you must include the X-API-KEY header to authorize API access.
Agreement Link
- The Customer must agree to Harbor’s Agreements before we can proceed with the KYC/AML verification process.
curl --location --request POST 'https://harbor-sandbox.owlpay.com/api/v1/customers' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header 'X-API-KEY: {{API_KEY}}' \
--header 'X-Idempotency-Key: {{Idempotency-Key}}' \
--data-raw '{
"type": "individual",
"first_name": "John",
"middle_name": "Michael",
"last_name": "Doe",
"email": "[email protected]",
"phone_country_code": "US",
"phone_number": "555-555-1234",
"birth_date": "1988-04-15",
"description": "A freelance writer who loves exploring national parks."
}'
The endpoint will return a response as shown below.
{
"data": {
"uuid": "{{customer_uuid}}",
"status": "deactivated",
"type": "individual",
"first_name": "John",
"middle_name": "Michael",
"last_name": "Doe",
"email": "[email protected]",
"phone_country_code": "US",
"phone_number": "555-555-1234",
"birth_date": null,
"has_signed_agreement": false,
"agreement_link": "https://harbor-sandbox.owlpay.com/agreements",
"verification_link": "https://harbor-sandbox.owlpay.com/gokyc",
"description": "A freelance writer who loves exploring national parks.",
"created_at": "2025-05-22T07:26:12+00:00",
"updated_at": "2025-05-22T07:26:12+00:00"
}
}
Note: You will receive a customer ID (cus_xxx). If you need to add bank accounts or other related information, this ID is required to make requests to the corresponding endpoints.
Share
agreement_link
andverification_link
to your customer
Customers
must agree to the agreement and complete the KYC form, which must be approved before proceeding to the next step.
- In Sandbox mode, approval is automatic and usually takes 1–2 minutes.
- In Production mode, the review process may take 1–2 business days in some cases.
Step 3: Move your first dollar (do an on/off ramp transfer)
The following is a quick reference to the on-ramp request and response. For detailed parameters, please see Transfer.
curl --location 'https://harbor-sandbox.owlpay.com/api/v1/transfer' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header 'X-API-KEY: {{API_KEY}}' \
--header 'X-Idempotency-Key: {{Idempotency-Key}}' \
--data-raw '{
"commission": {
"percentage": "0.01",
"amount": "1.00"
},
"source": {
"on_behalf_of": "{{YOUR_CUSTOMER_UUID}}",
"asset": "USD",
"amount": "100.00"
},
"destination": {
"asset": "USDC",
"chain": "ethereum",
"address": "0x4B20993Bc481177ec7E8f571ceCaE8A9e22C02db",
"transfer_purpose": "Salary",
"is_self_transfer": false,
"supporting_document": "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAQAAAC1HAwCAAAAC0lEQVR42mP8/x8AAwMCAOaCLP8AAAAASUVORK5CYII=" // Supporting document for the destination, providing supporting documents can effectively reduce the chances of being issued an RFI (Request For Information). Supported formats: png, jpg, jpeg, pdf, csv, docx, xlsx.
},
"application_transfer_uuid": "{{YOUR_APPLICATION_TRANSFER_UUID}}"
}'
Note: If you want to use a specific customer crypto address, include the customer_crypto_address_id field, and omit asset, chain, and address. Otherwise, you can specify the asset, blockchain network, and recipient address directly in the destination field.
See Customer's Crypto Address for more information.
curl --location 'https://harbor-sandbox.owlpay.com/api/v1/transfer' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header 'X-API-KEY: {{API_KEY}}' \
--header 'X-Idempotency-Key: {{Idempotency-Key}}' \
--data-raw '{
"commission": {
"percentage": "0.01",
"amount": "1.00"
},
"source": {
"on_behalf_of": "{{YOUR_CUSTOMER_UUID}}",
"asset": "USD",
"amount": "100.00"
},
"destination": {
"asset": "USDC",
"customer_crypto_address_id": "{{CUSTOMER_CRYPTO_ADDRESS_ID}}", // Optional, if you want to use a pre-created customer crypto address, you can provide the id here (If you input both, destination.address will be ignored)
"transfer_purpose": "Salary",
"is_self_transfer": false,
"supporting_document": "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAQAAAC1HAwCAAAAC0lEQVR42mP8/x8AAwMCAOaCLP8AAAAASUVORK5CYII=" // Supporting document for the destination, providing supporting documents can effectively reduce the chances of being issued an RFI (Request For Information). Supported formats: png, jpg, jpeg, pdf, csv, docx, xlsx.
},
"application_transfer_uuid": "{{YOUR_APPLICATION_TRANSFER_UUID}}"
}'
The endpoint will return a response as shown below.
{
"data": {
"uuid": "transfer_ewm6g1e0u6wRycKd7xeHqW0HaqLCV0CLj04xbn8d",
"status": "pending_customer_transfer_start",
"source": {
"on_behalf_of": "{{customer_uuid}}",
"asset": "USD",
"amount": "100.00"
},
"destination": {
"asset": "USDC",
"chain": "ethereum",
"address": "0x4B20993Bc481177ec7E8f571ceCaE8A9e22C02db",
"address_memo": null,
"is_self_transfer": false,
"beneficiary_name": null,
"amount": "98",
"transfer_purpose": "Salary"
},
"application_transfer_uuid": "{{YOUR_APPLICATION_TRANSFER_UUID}}",
"transfer_instructions": {
"account_number": "123456789012",
"routing_number": "987654321",
"bank_name": "FV Bank",
"bank_address": "1234 Maple Lane, Springfield, IL 62704, USA",
"account_holder_name": "OwlTing USA",
"narrative": "0297197683"
},
"commission": {
"percentage": "0.01",
"amount": "1.00"
},
"receipt": {
"initial_asset": "USD",
"initial_amount": "100.00",
"commission_fee": "2",
"harbor_fee": "0",
"final_asset": "USDC",
"final_amount": "98",
"exchange_rate": "1",
"transaction_hash": null
},
"created_at": "2025-05-22T07:59:57+00:00",
"updated_at": "2025-05-22T07:59:57+00:00"
}
}
Off-ramp (Sell USDC example)
curl --location 'https://harbor-sandbox.owlpay.com/api/v1/transfer' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header 'X-API-KEY: {{API_KEY}}' \
--header 'X-Idempotency-Key: {{Idempotency-Key}}' \
--data-raw '{
"commission": {
"percentage": "0.01",
"amount": "1.00"
},
"source": {
"on_behalf_of": "{{YOUR_CUSTOMER_UUID}}",
"chain": "ethereum",
"asset": "USDC",
"amount": "1000.00"
},
"destination": {
"asset": "USD",
"account_type": "checking",
"account_number": "123456789012",
"routing_number": "021000021",
"bank_name": "Test Bank USA",
"bank_country": "US",
"bank_state": "AL",
"bank_city": "CITY",
"bank_postal_code": "55123",
"bank_address": "123 Test Street, New York, NY 10001, USA",
"account_holder_name": "John Doe",
"residential_country_code": "US",
"residential_state": "DE",
"residential_city": "Wilmington",
"residential_address_1": "100 Market Street",
"residential_address_2": "Suite 200",
"transfer_purpose": "Salary", // required, describe the purpose of the transfer
"is_self_transfer": false, // required, Whether the transfer is self-transfer or not (if source and destination are different, please fill in false).
"supporting_document": "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAQAAAC1HAwCAAAAC0lEQVR42mP8/x8AAwMCAOaCLP8AAAAASUVORK5CYII=" // Supporting document for the destination, providing supporting documents can effectively reduce the chances of being issued an RFI (Request For Information). Supported formats: png, jpg, jpeg, pdf, csv, docx, xlsx.
},
"application_transfer_uuid": "{{YOUR_APPLICATION_TRANSFER_UUID}}"
}'
Note: If you want to use a specific customer bank account, include the customer_bank_account_id field, and omit bank information (account_number/routing_number/bank_name/bank_address/account_holder_name).
curl --location 'https://harbor-sandbox.owlpay.com/api/v1/transfer' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header 'X-API-KEY: {{API_KEY}}' \
--header 'X-Idempotency-Key: {{Idempotency-Key}}' \
--data-raw '{
"commission": {
"percentage": "0.01",
"amount": "1.00"
},
"source": {
"on_behalf_of": "{{YOUR_CUSTOMER_UUID}}",
"chain": "ethereum",
"asset": "USDC",
"amount": "1000.00"
},
"destination": {
"asset": "USD",
"customer_bank_account_id": "{{CUSTOMER_BANK_ACCOUNT_ID}}", // Optional, if you want to use a specific customer bank account, you can provide the id here (If you input both, customer_bank_account_id will be used first)
"transfer_purpose": "Salary",
"is_self_transfer": false,
"supporting_document": "data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAQAAAC1HAwCAAAAC0lEQVR42mP8/x8AAwMCAOaCLP8AAAAASUVORK5CYII=" // Supporting document for the destination, providing supporting documents can effectively reduce the chances of being issued an RFI (Request For Information). Supported formats: png, jpg, jpeg, pdf, csv, docx, xlsx.
},
"application_transfer_uuid": "{{YOUR_APPLICATION_TRANSFER_UUID}}"
}'
The endpoint will return a response as shown below.
{
"data": {
"uuid": "transfer_9r1k54ymg8xn9bleR88gWF4ItF4DGK4MR7ejuS6C",
"status": "pending_customer_transfer_start",
"source": {
"chain": "ethereum",
"on_behalf_of": "{{YOUR_CUSTOMER_UUID}}",
"asset": "USDC",
"amount": "1000.00"
},
"destination": {
"asset": "USD",
"account_number": "123456789012",
"routing_number": "021000021",
"iban": null,
"swift_code": null,
"bank_code": null,
"branch_code": null,
"bank_name": "Test Bank USA",
"bank_address": "123 Test Street, New York, NY 10001, USA",
"account_holder_name": "John Doe",
"account_holder_kana_name": null,
"residential_country_code": "US",
"residential_state": "DE",
"residential_city": "Wilmington",
"residential_address_1": "100 Market Street",
"residential_address_2": "Suite 200",
"is_self_transfer": false,
"beneficiary_name": "John Doe",
"amount": "989.00",
"transfer_purpose": "Salary"
},
"application_transfer_uuid": "{{YOUR_APPLICATION_TRANSFER_UUID}}",
"transfer_instructions": {
"instruction_chain": "ethereum",
"instruction_address": "0xbf2f9dd51e8f09408dea7334953398f06fff8959",
"instruction_memo": null
},
"commission": {
"percentage": "0.01",
"amount": "1.00"
},
"receipt": {
"initial_asset": "USDC",
"initial_amount": "1000.00",
"commission_fee": "11",
"harbor_fee": "0",
"final_asset": "USD",
"final_amount": "989.00",
"exchange_rate": "1",
"tracking_number": null
},
"created_at": "2025-05-22T08:06:27+00:00",
"updated_at": "2025-05-22T08:06:28+00:00"
}
}
Congratulations! You have the on/off-ramp deposit and withdrawal information. You can execute the payment according to the API response, and then your destination will receive the corresponding assets.
Updated about 1 month ago